27.5. Creating Ant Bundles
- An Ant recipe file named
deploy.xml
- Any associated application files. These application files can be anything; commonly, they fall into two categories:
- Archive files (JAR or ZIP files)
- Raw text configuration files, which can include tokens where users define the values when the bundle is deployed
Note
27.5.1. Supported Ant Versions
Software | Version |
---|---|
Ant |
|
Ant-Contrib |
|
27.5.2. Additional Ant References
- Apache Ant documentation main page
- Apache Ant documentation for the build file
27.5.3. Breakdown of an Ant Recipe
deploy.xml
.
Example 27.1. Simple Ant Recipe
<project>
root element and defined targets and tasks. The elements defined in the <rhq:bundle>
area pass metadata to the JBoss ON provisioning system when the project is built.
deploy.xml
file simply identifies the file as an an script and references the provisioning Ant elements.
<?xml version="1.0"?> <project name="test-bundle" default="main" xmlns:rhq="antlib:org.rhq.bundle">
<rhq:bundle>
element contains information about the specific version of the bundle (including, naturally enough, an optional version number).
<rhq:bundle name="Example App" version="2.4" description="an example bundle">
<rhq:bundle>
element that defines the name of the application. However, the bundle element contains all of the information about the application and, importantly, how the provisioning system should handle content contained in the application.
port
token defined in Section 27.5.5, “Using Templatized Configuration Files”, the <rhq:input-property>
element identifies it in the recipe. The name
argument is the input_field value in the token, the description
argument gives the field description used in the UI and the other arguments set whether the value is required, what its allowed syntax is, and any default values to supply. (This doesn't list the files which use tokens, only the tokens themselves.)
<rhq:input-property name="listener.port" description="This is where the product will listen for incoming messages" required="true" defaultValue="8080" type="integer"/>
<rhq:deployment-unit>
element. The entire application — its name, included ZIP or JAR files, configuration files, Ant targets — are all defined in the <rhq:deployment-unit>
parent element.
<rhq:deployment-unit>
directly. In this, the name is appserver
, and one preinstall target and one postinstall target are set.
<rhq:deployment-unit name="appserver" preinstallTarget="preinstall" postinstallTarget="postinstall" compliance="filesAndDirectories">
<rhq:deployment-unit>
element: the compliance
argument. Provisioning doesn't simply copy over files; as described in Section 27.1.3, “File Handling During Provisioning”, it remakes the directory to match what is in the bundle. If there are any existing files in the deployment directory when the bundle is first deployed, they are deleted by default. Setting compliance
to filesAndDirectories means that the provisioning process does not manage the deployment directory — meaning any existing files are left alone when the bundle is deployed.
/home/.rhqdeployments/
resourceID/backup
.
<rhq:file>
element. The name
is the name of the configuration file within the bundle, while the destinationFile
is the relative (to the deployment directory) path and filename of the file after it is deployed.
<rhq:file name="test-v2.properties" destinationFile="conf/test.properties" replace="true"/>
<rhq:archive>
element within the deployment-unit. The <rhq:archive>
element does three things:
- Identify the archive file by name.
- Define how to handle the archive. Simply put, it sets whether to copy the archive over to the destination and then leave it as-is, still as an archive, or whether to extract the archive once it is deployed. This is called exploding the archive. If an archive is exploded, then a postinstall task can be called to move or edit files, as necessary.
- Identify any files within the archive which contain tokens that need to be realized. This is a child element,
<rhq:fileset>
. This can use wildcards to include types of files or files within subdirectories or it can explicitly state which files to process.
<rhq:archive name="MyApp.zip" exploded="true"> <rhq:replace> <rhq:fileset> <include name="**/*.properties"/> </rhq:fileset> </rhq:replace> </rhq:archive>
<rhq:ignore>
element. In this case, any *.log
files within the logs/
directory are saved.
<rhq:ignore> <rhq:fileset> <include name="logs/*.log"/> </rhq:fileset> </rhq:ignore> </rhq:deployment-unit> </rhq:bundle>
<rhq:deployment-unit>
arguments. Most common Ant tasks are supported (as described in Section 27.5.4, “Using Ant Tasks”). This uses a preinstall task to print which directory the bundle is being deployed to and whether the operation was successful. The postinstall task prints a message when the deployment is complete.
<target name="main" /> <target name="preinstall"> <echo>Deploying Test Bundle v2.4 to ${rhq.deploy.dir}...</echo> <property name="preinstallTargetExecuted" value="true"/> <rhq:audit status="SUCCESS" action="Preinstall Notice" info="Preinstalling to ${rhq.deploy.dir}" message="Another optional message"> Some additional, optional details regarding the deployment of ${rhq.deploy.dir} </rhq:audit> </target> <target name="postinstall"> <echo>Done deploying Test Bundle v2.4 to ${rhq.deploy.dir}.</echo> <property name="postinstallTargetExecuted" value="true"/> </target> </project>
27.5.4. Using Ant Tasks
deploy.xml
file with some JBoss ON-specific elements. An Ant bundle distribution file supports more complex Ant configuration, including Ant tasks and targets.
27.5.4.1. Supported Ant Tasks
<antcall>
and <macrodef>
). This includes common commands like echo
, mkdir
, and touch
— whatever is required to deploy the content fully.
Important
<antcall>
element cannot be used with the Ant recipe. <antcall>
calls a target within the deploy.xml
file, which loops back to the file, which calls the <antcall>
task again, which calls the deploy.xml
file again. This creates an infinite loop.
<antcall>
, use the <ant>
task to reference a separate XML file which contains the custom Ant targets. This is described in Section 27.5.4.3, “Calling Ant Targets”.
Important
macrodef
call, and therefore macro definitions, are not supported with Ant bundles.
27.5.4.2. Using Default, Pre-Install, and Post-Install Targets
<project>
allows a default target, which is required by the provisioning system. This is a no-op because the Ant recipe mainly defines the metadata for and identifies files used by the provisioning process. Other operations aren't necessary. This target is required by Ant, even though it is a no-op target. Use pre- and post-install targets to perform tasks with the bundle before and after it is unpacked.
<target name="main" />
27.5.4.3. Calling Ant Targets
<antcall>
does not work in an Ant bundle recipe; it self-referentially calls the <rhq:bundle>
task in an infinite loop. However, it is possible to process tasks that are outside the default target. This can be done using pre- and post install targets (Section 27.5.4.2, “Using Default, Pre-Install, and Post-Install Targets”).
- In
deploy.xml
for the Ant recipe, add a<rhq:deployment-unit>
element which identifies the Ant target.<rhq:deployment-unit name="jar" postinstallTarget="myExampleCall">
- Then, define the target.
<target name="myExampleCall"> <ant antfile="another.xml" target="doSomething"> <property name="param1" value="111"></property> </ant> </target>
- Create a separate
another.xml
file in the same directory as thedeploy.xml
file. This file contains the Ant task.<?xml version="1.0"?> <project name="another" default="main"> <target name="doSomething"> <echo>inside doSomething. param1=${param1}</echo> </target> </project>
27.5.5. Using Templatized Configuration Files
Note
<rhq:input-property>
key in the Ant recipe. For examples, see Section 27.5.8.2, “rhq:input-property” and Example 27.1, “Simple Ant Recipe”.
input_field=@@property@@
port=@@listener.port@@
<rhq:input-property>
key in the Ant XML file.
<rhq:input-property name="listener.port" ... />
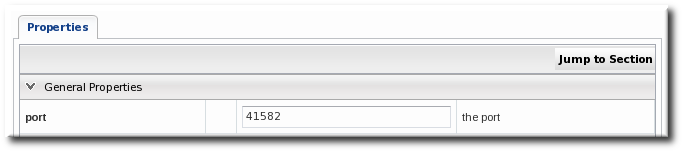
Figure 27.3. Port Token During Provisioning
Token | Description |
---|---|
rhq.deploy.dir | The directory location where the bundle will be installed. |
rhq.deploy.id | A unique ID assigned to the specific bundle deployment. |
rhq.deploy.name | The name of the bundle deployment. |
@@rhq.system.hostname@@
Token Name | Taken From... | Java API |
---|---|---|
rhq.system.hostname | Java API | SystemInfo.getHostname() |
rhq.system.os.name | Java API | SystemInfo.getOperatingSystemName() |
rhq.system.os.version | Java API | SystemInfo.getOperatingSystemVersion() |
rhq.system.os.type | Java API | SystemInfo.getOperatingSystemType().toString() |
rhq.system.architecture | Java API | SystemInfo.getSystemArchitecture() |
rhq.system.cpu.count | Java API | SystemInfo.getNumberOfCpus() |
rhq.system.interfaces.java.address | Java API | InetAddress.getByName(SystemInfo.getHostname()).getHostAddress() |
rhq.system.interfaces.network_adapter_name.mac | Java API | NetworkAdapterInfo.getMacAddress() |
rhq.system.interfaces.network_adapter_name.type | Java API | NetworkAdapterInfo.getType() |
rhq.system.interfaces.network_adapter_name.flags | Java API | NetworkAdapterInfo.getAllFlags() |
rhq.system.interfaces.network_adapter_name.address | Java API | NetworkAdapterInfo.getUnicastAddresses().get(0).getHostAddress() |
rhq.system.interfaces.network_adapter_name.multicast.address | Java API | NetworkAdapterInfo.getMulticastAddresses().get(0).getHostAddress() |
rhq.system.sysprop.java.io.tmpdir | Java system property | |
rhq.system.sysprop.file.separator | Java system property | |
rhq.system.sysprop.line.separator | Java system property | |
rhq.system.sysprop.path.separator | Java system property | |
rhq.system.sysprop.java.home | Java system property | |
rhq.system.sysprop.java.version | Java system property | |
rhq.system.sysprop.user.timezone | Java system property | |
rhq.system.sysprop.user.region | Java system property | |
rhq.system.sysprop.user.country | Java system property | |
rhq.system.sysprop.user.language | Java system property |
27.5.6. Processing JBoss ON Properties and Ant Properties
<rhq:input-property>
tags. These are input properties; at the time the bundle is deployed, the server parses the configuration in the file and prompts for any input properties. It then deploys the bundle using those user-defined values.
<property>
tags — but in a more limited way than a normal Ant build system.
<rhq:bundle>
element for the archive file <rhq:archive>
and any other associated content.
27.5.7. Limits and Considerations for Ant Recipes
27.5.7.1. Unsupported Ant Tasks
<antcall>
(instead, use<ant>
to reference a separate XML file in the bundle which contains the Ant targets)<macrodef>
and macro definitions
27.5.7.2. Symlinks
java.util.zip
) included for the bundling system does not support symbolic links. Therefore, bundle recipes and configuration files cannot use symlinks.
<untar src="abc.tar.gz" compression="gzip" dest="somedirectory"/>
27.5.7.3. WARNING: The Managed (Target) Directory and Overwriting or Saving Files
- The file in the current directory is also in the bundle. In this case, the bundle file always overwrites the current file. (There is one exception to this. If the file in the bundle has not been updated and is the same version as the local file, but the local file has modifications. In that case, the local file is preserved.)
- The file in the current directory does not exist in the bundle. In that case, the bundle deletes the file in the current directory.
compliance
, <rhq:ignore>
, and cleanDeployment
.
All of the information about the application being deployed is defined in the <rhq:deployment-unit>
element in a bundle recipe. The compliance
attribute on the <rhq:deployment-unit>
element sets how the provisioning process should handle existing files in the deployment directory.
Note
/home/.rhqdeployments/
resourceID/backup
.
compliance
attribute applies to both the initial deployment and upgrade operations, so this can be used to preserve files that may exist in a directory before a bundle is ever deployed.
Note
There can be files that are used or created by an application, apart from the bundle, which need to be preserved after a bundle deployment. This can include things like log files, instance-specific configuration files, or user-supplied content like images. These files can be ignored during the provisioning process, which preserves the files instead of removing them.
<rhq:ignore>
element and list the directories or files to preserve.
<rhq:ignore> <rhq:fileset> <include name="logs/*.log"/> </rhq:fileset> </rhq:ignore>
<rhq:ignore>
element only applies when bundles are updated; it does not apply when a bundle is initially provisioned.
<rhq:ignore>
element only applies to file that exist outside the bundle. Any files that are in the bundle will overwrite any corresponding files in the deployment directory, even if they are specified in the <rhq:ignore>
element.
Both compliance
and <rhq:ignore>
are set in the recipe. At the time that the bundle is actually provisioned, there is an option to run a clean deployment. The clean deployment option deletes everything in the deployment directory and provisions the bundle in a clean directory, regardless of the compliance
and <rhq:ignore>
settings in the recipe.
27.5.8. A Reference of JBoss ON Ant Recipe Elements
27.5.8.1. rhq:bundle
Attribute Description Optional or Required name The name given to the bundle. Required version The version string for this specific bundle. Bundles can have the same name, but each bundle of that name must have a unique version string. These version strings normally conform to an OSGi style of versioning, such as 1.0
or 1.2.FINAL
. Required description A readable description of this specific bundle version. Optional
<rhq:bundle name="example" version="1.0" description="an example bundle">
27.5.8.2. rhq:input-property
Note
<rhq:input-property>
definition.
Attribute Description Optional or Required name The name of the user-defined property. Within the recipe, this property can be referred to by this name, in the format ${
property_name}
. Required description A readable description of the property. This is the text string displayed in the JBoss ON bundle UI when the bundle is deployed. Required type Sets the syntax accepted for the user-defined value. There are several different options:
Required required Sets whether the property is required or optional for configuration. The default value is false
, which means the property is optional. If this argument isn't given, then it is assumed that the property is optional. Optional defaultValue Gives a value for the property to use if the user does not define a value when the bundle is deployed. Optional
<rhq:input-property name="listener.port" description="This is where the product will listen for incoming messages" required="true" defaultValue="8080" type="integer"/>
27.5.8.3. rhq:deployment-unit
<rhq:deployment-unit>
element in a bundle recipe.
Attribute Description Optional or Required name The name of the application. Required compliance Sets whether JBoss ON should manage all files in the top root directory (deployment directory) where the bundle is deployed. If filesAndDirectories, any unrelated files found in the top deployment directory (files not included in the bundle) are ignored and will not be overwritten or removed when future bundle updates are deployed. The default is full, which means that the provisioning process manages all files and directories and removes or overwrites anything not in the bundle.
/home/.rhqdeployments/
resourceID/backup
.
Optional preinstallTarget An Ant target that is invoked before the deployment unit is installed. Optional postinstallTarget An Ant target that is invoked after the deployment unit is installed. Optional
<rhq:deployment-unit name="appserver" preinstallTarget="preinstall" postinstallTarget="postinstall">
27.5.8.4. rhq:archive
Note
${}
property definition.
Important Attribute Description Optional or Required name The filename of the archive file to include in the bundle.
name
must contain the relative path to the location of the archive file in the ZIP file.
Required exploded Sets whether the archive's contents will be extracted and stored into the bundle destination directory (true) or whether to store the files in the same relative directory as is given in the name
attribute (false). If the files are exploded, they are extracted starting in the deployment directory. Post-install targets can be used to move files after they have been extracted. Optional
<rhq:archive name="file.zip"> <rhq:replace> <rhq:fileset> <include name="**/*.properties"/> </rhq:fileset> </rhq:replace> </rhq:archive>
27.5.8.5. rhq:url-archive
rhq:archive
except that the server accesses the archive over the network rather than including the archive directly in the bundle distribution file.
Note Note Attribute Description Optional or Required url Gives the URL to the location of the archive file. The archive is downloaded and installed in the deployment directory.
Required exploded If true, the archive's contents will be extracted and stored into the bundle destination directory; if false, the zip file will be compressed and stored in the top level destination directory.
Optional
<rhq:url-archive url="http://server.example.com/apps/files/archive.zip"> <rhq:replace> <rhq:fileset> <include name="**/*.properties"/> </rhq:fileset> </rhq:replace> </rhq:url-archive>
27.5.8.6. rhq:file
<rhq:file>
element calls out files that require processing before they should be copied to the destination. The attributes on the <rhq:file>
element set the name of the raw file in the bundle distribution ZIP file and the name of the target file that it should be copied to.
Note
${}
property definition.
Important Note Attribute Description Optional or Required name The name of the raw configuration file.
name
must contain the relative path to the location of the file within the ZIP file.
Required destinationFile The full path and filename for the file on the destination resource. Relative paths must be relative to the final deployment directory (defined in the rhq.deploy.dir
parameter when the bundle is deployed). It is also possible to use absolute paths, as long as both the directory and the filename are specified.
destinationDir
attribute is used, the destinationFile
attribute cannot be used.
Required, unless destinationDir is used destinationDir The directory where this file is to be copied. If this is a relative path, it is relative to the deployment directory given by the user when the bundle is deployed. If this is an absolute path, that is the location on the filesystem where the file will be copied.
name
attribute.
destinationFile
attribute is used, the destinationDir
attribute cannot be used.
Required, unless destinationFile is used replace Indicates whether the file is templatized and requires additional processing to realize the token values. Required
<rhq:file name="test-v2.properties" destinationFile="subdir/test.properties" replace="true"/>
destinationDir
nor the destinationFile
attribute is used, then the raw file is placed in the same location under the deployment directory as its location in the bundle distribution.
27.5.8.7. rhq:url-file
rhq:file
, contains the information to identify and process configuration files for the application which have token values that must be realized. This option specifies a remote file which is downloaded from the given URL, rather than being included in the bundle archive.
Note Note Attribute Description Optional or Required url Gives the URL to the templatized file. The file is downloaded and installed in the deployment directory.
Required destinationFile The full path and filename for the file on the destination resource. Relative paths must be relative to the final deployment directory (defined in the rhq.deploy.dir
parameter when the bundle is deployed). It is also possible to use absolute paths, as long as both the directory and the filename are specified.
destinationDir
attribute is used, the destinationFile
attribute cannot be used.
Required, unless destinationDir is used destinationDir The directory where this file is to be copied. If this is a relative path, it is relative to the deployment directory given by the user when the bundle is deployed. If this is an absolute path, that is the location on the filesystem where the file will be copied.
name
attribute.
destinationFile
attribute is used, the destinationDir
attribute cannot be used.
Required, unless destinationFile is used replace Indicates whether the file is templatized and requires additional processing to realize the token values. Required
<rhq:url-file url="http://server.example.com/apps/files/test.conf" destinationFile="subdir/test.properties" replace="true"/>
destinationDir
nor the destinationFile
attribute is used, then the raw file is placed in the same location under the deployment directory as its location in the bundle distribution.
27.5.8.8. rhq:audit
rhq:audit
configuration sends information to the server about the additional processing steps and their results.
Attribute Description Optional or Required status The status of the processing. The possible values are SUCCESS, WARN, and FAILURE. The default is SUCCESS. Optional action The name of the processing step. Required info A short summary of what the action is doing, such as the name of the target of the action or an affected filename. Optional message A brief text string which provides additional information about the action. Optional
<rhq:audit status="SUCCESS" action="Preinstall Notice" info="Preinstalling to ${rhq.deploy.dir}" message="Another optional message"> Some additional, optional details regarding the deployment of ${rhq.deploy.dir} </rhq:audit>
27.5.8.9. rhq:replace
<rhq:fileset>
elements, contained in the archive which need to have token values realized when the archive is deployed.
<rhq:replace>
element.
<rhq:archive name="file.zip"> <rhq:replace> <rhq:fileset> <include name="**/*.properties"/> </rhq:fileset> </rhq:replace> </rhq:archive>
27.5.8.10. rhq:ignore
<rhq:replace>
, contains a list of files or directories in the instance to save.
Note
Important
/opt/myapp
and Bundle B to /opt/myapp/webapp1
).
<rhq:ignore> <rhq:fileset> <include name="logs/*.log"/> </rhq:fileset> </rhq:ignore>
27.5.8.11. rhq:fileset
<rhq:replace>
and <rhq:ignore>
— define file lists in either the archive file or the deployment directory. This element contains the list of files.
Child Element Description <include name=filename /> The filename of the file. For <rhq:replace>
, this is a file within the archive (JAR or ZIP) file which is templatized and must have its token values realized. For <rhq:ignore>
, this is a file in the application's deployment directory which should be ignored and preserved when the bundle is upgraded.
<rhq:replace> <rhq:fileset> <include name="**/*.properties"/> </rhq:fileset> </rhq:replace>
27.5.8.12. rhq:system-service
Attribute Description Optional or Required name The name of the script. Required scriptFile The filename of the script. If the script file is packaged with the Ant recipe file inside the bundle distribution ZIP file, then the scriptFile
must contain the relative path to the location of the file in the ZIP file. Required configFile The name of any configuration or properties file used by the script. If the configuration file is packaged with the Ant recipe file inside the bundle distribution ZIP file, then the configFile
must contain the relative path to the location of the file in the ZIP file. Optional overwriteScript Sets whether to overwrite any existing init file to configure the application as a system service. Optional startLevels Sets the run level for the application service. Optional startPriority Sets the start order or priority for the application service. Optional stopPriority Sets the stop order or priority for the application service. Optional
<rhq:system-service name="example-bundle-init" scriptFile="example-init-script" configFile="example-init-config" overwriteScript="true" startLevels="3,4,5" startPriority="80" stopPriority="20"/>